If you understand basic C# and want to understand how block chain works. Here is atoy version of blockchain written in C#.
In here, a block stands for one transaction. You can see how a block is being constructed and how a block relates to its previous block.
The most interesting part is you can tell how a block can be mined. Check out the Mine() method.
It is really about just finding out the hash matching certain pattern. In here, it is about matching certain number of leading 0s in the final hash.
class Block { public int Index { get; set; } public string PreviousHash { get; set; } public string Timestamp { get; set; } public string Data { get; set; } public string Hash { get; set; } int _salt; public Block(int index, string timestamp, string data, string previousHash = "") { this.Index = index; this.PreviousHash = previousHash; this.Timestamp = timestamp; this.Data = data; this.Hash = this.CalculateHash(); this._salt = 0; } // Create the hash of the current block. public string CalculateHash() { var hash = SHA256_hash(this.Index + this.PreviousHash + this.Timestamp + this.Data + this._salt); return hash; } // This is how the mining works! public void Mine(int difficulty) { // You mined successfully if you found a hash with certain number of leading '0's // difficulty defines the number of '0's required // e.g. if difficulty is 2, if you found a hash like 00338500000x..., it means you mined it. while (this.Hash.Substring(0, difficulty) != new String('0', difficulty)) { this._salt++; this.Hash = this.CalculateHash(); Console.WriteLine("Mining:" + this.Hash); } Console.WriteLine("Block has been mined: " + this.Hash); } // Create a hash string from stirng static string SHA256_hash(string value) { var sb = new StringBuilder(); using (SHA256 hash = SHA256Managed.Create()) { var enc = Encoding.UTF8; var result = hash.ComputeHash(enc.GetBytes(value)); foreach (var b in result) sb.Append(b.ToString("x2")); } return sb.ToString(); } }
A Blockchain contains numbers of blocks. Its main task is to build the chain and validate it.
Check out ValidateChain() method.
class Blockchain { // a chain has many blocks List<Block> _chain; public int Difficulty { get; set; } public Blockchain() { this._chain = new List<Block>(); this._chain.Add(CreateGenesisBlock()); this.Difficulty = 2; } Block CreateGenesisBlock() { return new Block(0, "01/12/2017", "Genesis block", ""); } public Block GetLatestBlock() { return this._chain.Last(); } // Once a block is mined, add it to the block public void AddBlock(Block newBlock) { newBlock.PreviousHash = this.GetLatestBlock().Hash; newBlock.Mine(this.Difficulty); this._chain.Add(newBlock); } public void ValidateChain() { for (var i = 1; i < this._chain.Count; i++) { var currentBlock = this._chain[i]; var previousBlock = this._chain[i - 1]; // Check if the current block hash is consistent with the hash calculated if (currentBlock.Hash != currentBlock.CalculateHash()) { throw new Exception("Chain is not valid! Current hash is incorrect!"); } // Check if the Previous hash match the hash of previous block if (currentBlock.PreviousHash != previousBlock.Hash) { throw new Exception("Chain is not valid! PreviousHash isn't pointing to the previous block's hash!"); } } } }
Download the source code on GitHub
What is Industry Connect?
Industry Connect is an IT/Software Career Launchpad that has everything you need to kick-start your IT/software career in AU, NZ, UK, IE, SG, HK, IN, ID, VN & PH.
We have been helping career changers, recent IT graduates and people with career gap to start their IT/software careers.
Over the years, we have helped hundreds kick-start an IT/software career. (Verifiable evidences are available on this website)
OUR CORE
We are an innovative software training school backed by global/local software companies. Our ecosystem is an incubation process that supports our participants until they launch a tech career.
OUR PROGRAMMES
Our Job-Ready training programmes focus on,
PROGRAMME STRUCTURE
You can join us via Zoom (live face-to-face meeting) remotely from anywhere and "download" the valuable knowledge & experiences from our tech experts across different tech centres.
Industry Connect's Ecosystem
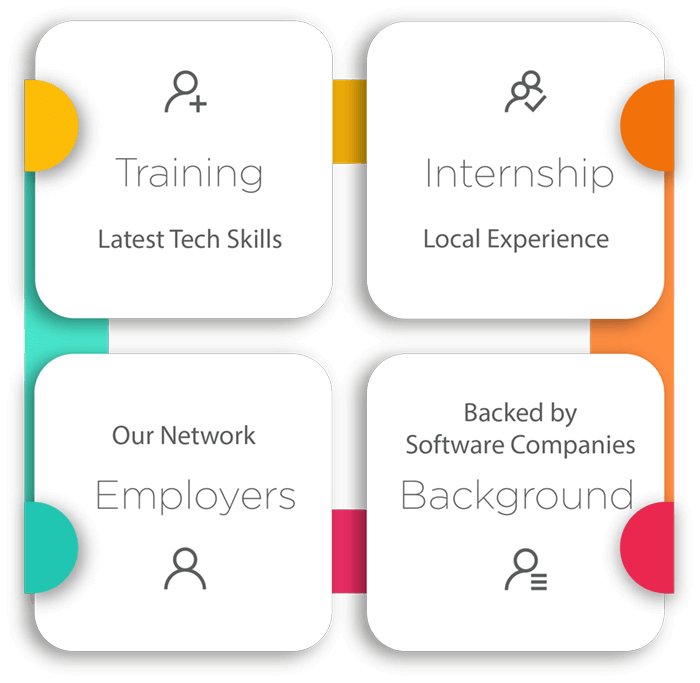
We have been helping career changers, recent IT graduates and people with career gap to start their IT/software careers.
Over the years, we have helped hundreds kick-start an IT/software career. (Verifiable evidences are available on this website)
OUR CORE
We are an innovative software training school backed by global/local software companies. Our ecosystem is an incubation process that supports our participants until they launch a tech career.
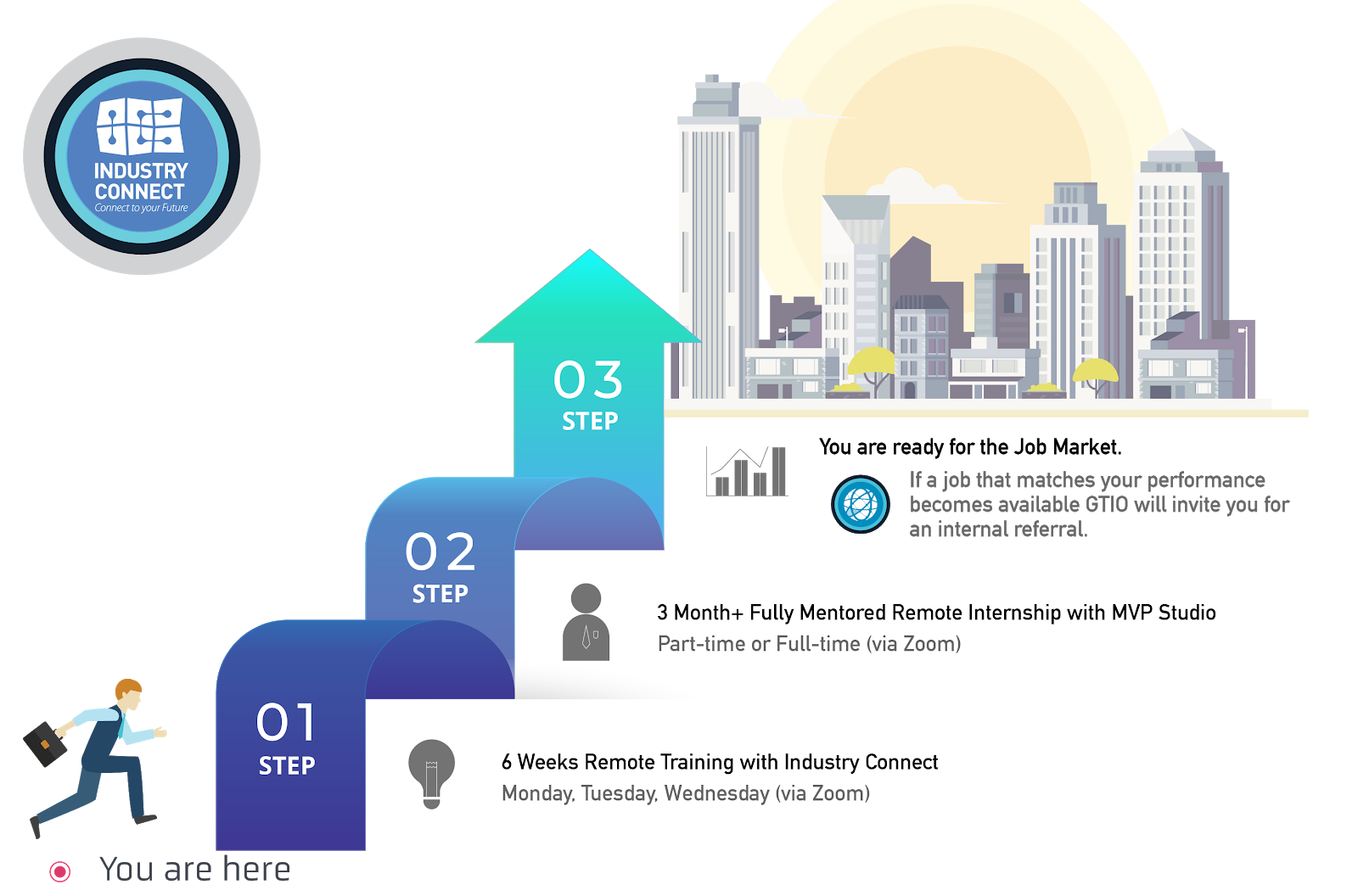
OUR PROGRAMMES
Our Job-Ready training programmes focus on,
- Software Development
- Business Intelligence (or Data Analyst)
- Test Analyst
PROGRAMME STRUCTURE
- Six Weeks Training Classes (Practical Tech Skills)
- Three Months (or more) Structured Internship on Large Commercial-Scale Projects (Learning enhanced, flexible Hours)
- Employer Network (job analysis and internal referral if goals are met)
You can join us via Zoom (live face-to-face meeting) remotely from anywhere and "download" the valuable knowledge & experiences from our tech experts across different tech centres.